LOOM Frequently Asked Questions
I get a System.MethodAccessException
when I'm trying to interweave an aspect
This indicates that either you're aspect or your target class is not marked as public.
Why Advice.AfterThrowing
eats my exceptions?
If you use the Advice.AfterThrowing
join point attribute, then keep in mind that the interwoven code will eat the exception. To avoid this, re-throw the exception from your aspect code:
[Call(Advice.AfterThrowing)] public void f([JPException] Exception e) /// ... your code throw e; // rethrow to keep the exception alive
3. I am confused about the call order when I interweave more then one aspect
Loom interweaves the aspects in the following order
- all aspects defined as assembly attribute
- all aspects defined as module attribute
- all aspects defined as class attributes
- all aspects given in the aspects parameter of
Weaver.CreateInstance
in reverse order
This has the following implications when you call a interwoven method:
Advice.Before
: LICFAdvice.Around
: LICFInvoke.AfterXXX
: FICF
LICF = Last Interwoven Called First: the aspect which is the first in the aspects parameter will be called first. The first assembly attribute will be the last one.
FICF = First Interwoven Called First: the first aspect defined as assembly attribute will be called first and the first parameter of the aspects parameter will be called last.
If my aspect has more than one matching aspect method, in which order they will be interwoven?
If both methods belong to the same join point category, then it is the same order as they declared in the aspect class. Otherwise it is the following order:
- all
Advice.Before
methods, if there exist one - the
Advice.Around
method, if there is one - all
Advice.AfterReturning
andAdvice.AfterThrowing
methods, if there exist one - all
Advice.After
methods, if there exist one
I've defined an aspect method but loom won't interweave this
Be sure that the target class method is either virtual or defined via an interface. If it is a virtual method it has to be at least protected. Your aspect method on the other hand should be declared as public. If you interweave interface methods, you have to cast your interwoven object to the appropriate interface before you call the interwoven method.
How many aspect instances are created, during my interweaving process?
To take control over the creation of aspect instances, you have to use the InstanceCreation
attribute. The attribute can be used to annotate aspect classes only. There are three different creation types, depending on the CreationType
you are using:
This UML model shows the classes of an InstanceCreation example.
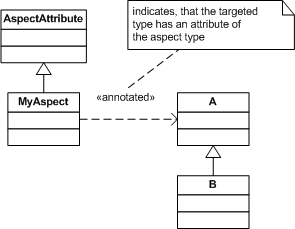
using InstanceCreation(PerInstance)
(default):
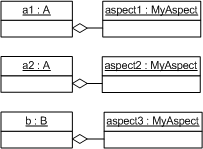
using InstanceCreation(PerClass)
:
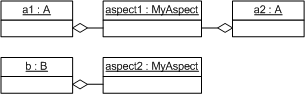
using InstanceCreation(PerAttribute)
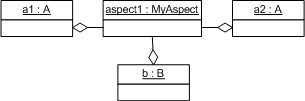